In this article, we are going to create Rest API using NodeJs, Express, and MongoDB. In the first phase, we will describe what do we mean by REST API.
Definition of Restful API
REST is an acronym for “REpresentational State Transfer”. Like any other architectural style, Rest also has its guiding protocol, which consists of SIX guidings constraints.
Guiding principles of REST
- Stateless – Each request from the client should contain all necessary information which is essential to understand the request.
- Cacheable – If the user request is implicitly or explicitly marked as cacheable. Then it has the right to reuse the response data for equivalent recommendations(request).
- Client-server – By using the REST API, we have separated the client and server concepts. It will improve the scalability of application and reusability.
- User Interface – The overall system architecture is improved (Simplified) by approaching the REST API.
For more information, you can visit.
In this article, we are going to create API to demonstrate the CRUD operation. We will use NodeJs, Express and MongoDB.
Step 1: Initialization of project
First, we need to create a project folder. After making the project folder, we need to initialize the application. We need to create the package.json file which will hold all the metadata for the applications. We need to execute the following command
npm init -y
The above-given command will create a package.json file
Step 2: Install the necessary
In this section, we will install all the required dependencies of the project. We will run the following command to install the dependencies
touch app.js
npm install express mongoose body-parser --save
Using the given commands we have created a file app.js which will be the main entry point of the application. After that, we have installed the few dependencies that are essential to run our application.
These are the dependencies.
- MongoDB – This module is used to communicate to the MongoDB database.
We are using the mongoose npm package to interact with the MongoDB database. We are going to connect to the MongoDB database using the free service of MongoDB official website(www.mongodb.com). - Express – NodeJs framework
- Body Parser – It will allow us to handle the request body with express.
Step 3: Setup the database
Login to www.mongodb.com there we can create our account fo free. We will use this service to connect to the MongoDB database. After login, we need to set up our default language we can change it later as per our requirement. I have set Javascript as per now because we are using NodeJs.

After selecting the default language, we need to continue. Next step, is to create the shared cluster it is absolutely free.

Next, we need to select the cloud server and region. I have chosen AWS and the nearest data center as per your current location. It will take some time to complete the process. Next, we need to create the user and collection name. The user is used to connecting to the database from the application.

Next, step is to click on the connect option that you got on the next page. There we need to add the username and password that we will use to connect to the application. Please store that username and password we will need that while connecting to the application. After that we need to choose a connection method.

In that, we need to choose an option for the connection method. We will opt for “Connect your application.”

After selecting the option “Connect your application.” we will get a window there, we need to copy the connection string. In the copy connection string, replace username, password, and database name as we have created.

Step 4: Implementing the CRUD functionality using NodeJs, Express and MongoDB
In earlier steps, we have initialized the application and created the basic structure of the project.
We are going to store the user information for demonstrating the CRUD functionality using NodeJs, Express and MongoDB. We are going to store the following details for the user.
- Username
- Contact
- City
- Gender
Let’s structure our project first app.js will be our entry file into the application. We will divide code into the three main parts in a big project we need to go into the more dipper level. The folder structure will look like this, We have created a separate folder structure for the components in the project like the controller, model, and routes.

- Model: In this, we will define the structure of the collection that we are going to create.
- Controller: In this, we will perform the application functionality.
- Routes: In this, we will make the route by which the user will interact with the API.
We will start with the Model file that is in the model folder i.e user.js.
In that first, we have required the mongoose which we have installed at the beginning of the project. It will use to initialize the model for the collection. In this, we have defined the value that we want to store in the database like username, city, email, contact and gender.
//mongoose to connect to the MongoDb
const mongoose = require('mongoose');
// simple Schema
const UserSchemaInfo = new mongoose.Schema({
name: {
type: String,
required: true,
minlength: 4,
maxlength: 60
},
email: {
type: String,
required: true,
minlength: 6,
maxlength:255,
unique: true
},
contact: {
type: String,
required: true,
minlength: 11,
maxlength:100
},
city: {
type: String,
minlength: 6,
maxlength:100
},
gender: String,
create_date:{
type:Date,
default: Date.now
}
});
const Userinfo = mongoose.model('User', UserSchemaInfo);
exports.UserInfo = Userinfo;
Second Part will be the controller in this file we have defined all the operation that we are going to perform on the user collection, i.e. Create, Read, Update and delete.
In this first, we have imported the required model file. After that first operation “exports.index” in this, we have returned all the saved user in the database. In the second operation “exports.userNew” we have created the new user. Here we are merely inserting the data. We can check like the same user cannot be added twice etc. as per our requirement.
In the third function, we are finding the user by the user id and returning the fetched user.
In the fourth function, we are deleting the user by the provided user id.
In the last function, we are updating the user information provided by the input.
// user controller we will here perform all the action
// import the the cuser model
const { UserInfo } = require('../model/user');
// API to handle the Index Action or we can say that
exports.index = function ( req, res ) {
UserInfo.find(function (err, users){
if(err){
res.json({
status: 'error',
message: err
})
}
res.json({
status:"success",
message: 'User information sucessfully',
data: users
});
});
};
// API to handle the creation of the user
exports.userNew = function (req, res) {
var user = new UserInfo();
user.name = req.body.name;
user.email = req.body.email;
user.contact = req.body.contact;
user.city = req.body.city;
user.gender = req.body.gender;
// use to save the user in the databases
user.save( function (err) {
if(err){
res.json({
status: 'error',
message: err
})
}
// if there is no error return the user detail
res.json({
message: "User created successfully",
data: user
});
})
};
// to retrieve the user info based on the user ID
exports.userInfoById = function(req, res){
UserInfo.findById(req.params.user_id, function(err, userData){
if (err){
res.json({
status: 'User not found',
message: err
});
}
res.json({
message: "user info details by id",
data: userData
});
});
};
// Delete the user
exports.deleteUser = function (req, res){
UserInfo.remove({
_id: req.params.user_id
}, function (err, user) {
if (err){
// if there is no user found to delete the user
res.json({
status: 'User is not deleted',
message: err
});
}
// if user is deleted successfully
res.json({
message:"User deleted successfully",
data: user
});
});
};
// Function to update the user
exports.updateUser = function (req,res){
// first we will find the user existor not if user exist we will update user
UserInfo.findById(req.params.user_id, function(err, user) {
if (err){
res.json({
status: 'User not found',
message: err
});
}
user.name = req.body.name;
user.email = req.body.email;
user.contact = req.body.contact;
user.city = req.body.city;
user.gender = req.body.gender;
user.save(function (err) {
if(err){
res.json({
status: 'User not updated',
message: err
});
}
res.json({
message: 'User updated successfully',
data: user
});
});
});
};
The third part will be the routes in that file. We will define how the user will interact with the application by using that endpoint.
We will implement the following endpoints
- GET /api/userInfo/users list all contacts
- POST /api/userInfo/user create new contact
- GET /api/userInfo/user/{user_id} retrieve a single contact
- PUT /api/userInfo/user/{user_id} update a single contact
- DELETE /api/userInfo/user/user_id} delete a single contact
// intialize the express router for the user
const router = require('express').Router();
// default API response
router.get('/', function(req, res) {
res.json({
status: 'API is initialize the working',
message: 'user default REST API'
});
});
// import the user controller
const userController = require('../controller/userController');
// User routes
router.route('/users')
.get(userController.index)
.post(userController.userNew);
// routes to get the user info based on the user id
router.route('/user/:user_id')
.get(userController.userInfoById)
.patch(userController.updateUser)
.put(userController.updateUser)
.delete(userController.deleteUser);
// exporting the user API routes
module.exports = router;
The last file will be our entry file to the application, i.e. app.js. In this firstly, we have required the express, i.e. node web framework. Second is body-parser it is node js middleware to handle, available under the req.body property. If you want to learn more about middleware in the NodeJs, please visit NodeJs Middleware. After that, we have required our routes file. We have connected to our database. We have added the routes for the user. By this, we have completed our implementation. Let’s try the different routes to perform CRUD operations.
// import the express
const express = require("express");
// import the body Parser
const bodyParser = require('body-parser');
// import the mongoose to connect to the MongoDB
const mongoose = require("mongoose");
// import the user routes
const userRoutes = require("./routes/userRoutes");
const port = process.env.PORT || 9001;
const app = express();
// configure bodyparser to handle the post request
app.use(bodyParser.urlencoded({
extended:true
}));
// use body parser
app.use(bodyParser.json());
//connection to the moongoose
const connection_url = 'mongodb+srv://adminTest:admin@123@cluster0.qutfh.mongodb.net/userInfo?retryWrites=true&w=majority'
mongoose.connect(connection_url, {
useCreateIndex:true,
useNewUrlParser: true,
useUnifiedTopology: true
}).then(() => console.log('connected to MongoDb'))
.catch(err => console.error("could not connect to mongodb"));
// URL for the default URL
app.get('/', (req,res) => res.send(' API request with express'));
// adding the route for User
app.use("/api/userInfo", userRoutes);
app.listen(port, () =>
console.log(`listening on port ${port}`));
Now we will run the application by executing this command in the terminal
node app.js
Now we will use all the routes that we have created (CRUD).
- Create . In this we will create a new user by using PostMan. Our URL will be “localhost:9001/api/userInfo/users”. The method will be POST for creation operation.

2. Read: We can fetch one user detail by user_id or we can get all the user’s information lets to try this URL “localhost:9001/api/userInfo/users”. The method will be ‘GET’ for retrieving the information for the database.

3. Update: In this we will update the user information. URL will be “localhost:9001/api/userInfo/user/5f94f0be5c89ef36023fba28”. The method will be “PUT” for updating the user in the database.
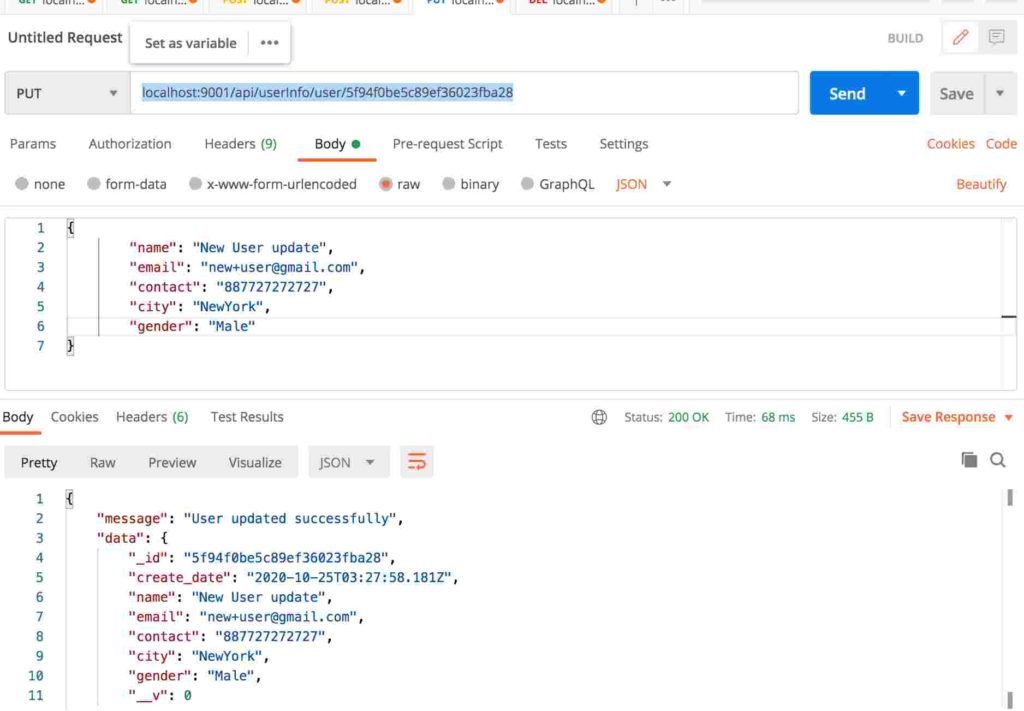
4. Delete: In this section, we will delete the user by the user_id provide by the user. URL will be
“localhost:9001/api/userInfo/user/5f94f0e45c89ef36023fba29”. The method will be “DELETE”
for deleting the user information from the database.

Conclusion
In this tutorial, we are trying to learn and develop a custom API and connect to the MongoDB and fetch or manipulate the data. However, this is a very basic project to understand the CRUD functionality. You can add more features like security, validation of data. Please try in your side if you find any problem I am here to help you please free to connect me.
1 thought on “How to Create a Simple Restful API using NodeJs, ExpressJs, And MongoDB”
Comments are closed.